React is a famous user interface (UI) JavaScript library. You can use React library to create different kinds of web apps ranging from social media apps, eCommerce platforms, blogs, single-page applications, content management systems (CMS), Dashboards, and data visualizations, to mention a few.
Developers can extend the functionality of React apps using various libraries and frameworks. Such libraries can be grouped into different classes; Drag-and-Drop is a library category that is quite popular.
Drag and Drop functionality is a UI interaction that allows a user to click/ pick an element on the screen, drag it and drop it on another component. A perfect example of this functionality is rearranging items in a list by dragging and dropping items to your desired location.
You can also use Drag-and-Drop functionality in the following cases;
- File upload: Users can drag and drop files instead of scrolling through their machines to select and upload files.
- Kanban boards: You can create a dashboard where users can drag and drop items depending on activity levels or stages.
- Image galleries: You can have an image gallery where users can upload and rearrange images.
- Widgets: Users can customize the app’s appearance by dragging and dropping widgets.
- Shopping cart: Users can click on an item, drag it and drop it into the cart.
A React Drag and Drop library is a set of pre-built components that allow developers to implement Drag and Drop functionality in React applications.
These libraries come with reusable components, allowing developers to create elements that can be dragged and dropped. The libraries handle different events such as drag start, drag enter, drag leave, and drop.
How Drag and Drop libraries will help in better UI interactions
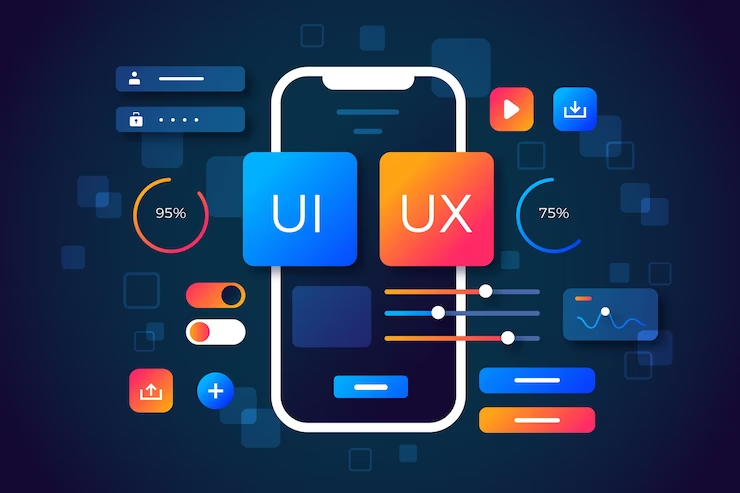
- Enhanced user experience: The drag-and-drop functionality is an intuitive approach for users to interact with an application. The option of dragging and dropping items instead of inputting them manually provides an interactive and seamless interaction.
- Simplified workflows: Instead of uploading files from different locations of your computer to an application, you can simply drag and drop them.
- Increased productivity: Drag-and-drop functionality saves time, making users more productive.
- Suitable for mobile devices: Mobile devices like smartphones and tablets have limited screen space. Users mostly rely on gestures for navigation, which makes drag-and-drop an awesome addition.
- Provides engaging interfaces: Drag-and-drop functionality can add visual appeal to your app’s user interface. You can add animations that provide feedback or describe actions as users drag and drop items on the app.
These are the best Drag and Drop React libraries:
React DnD
React DnD is a set of React utilities for building complex drag-and-drop interfaces. This library is perfect for creating apps similar to Trello and Storify, where drag-and-drop functionality also involves data transfer.
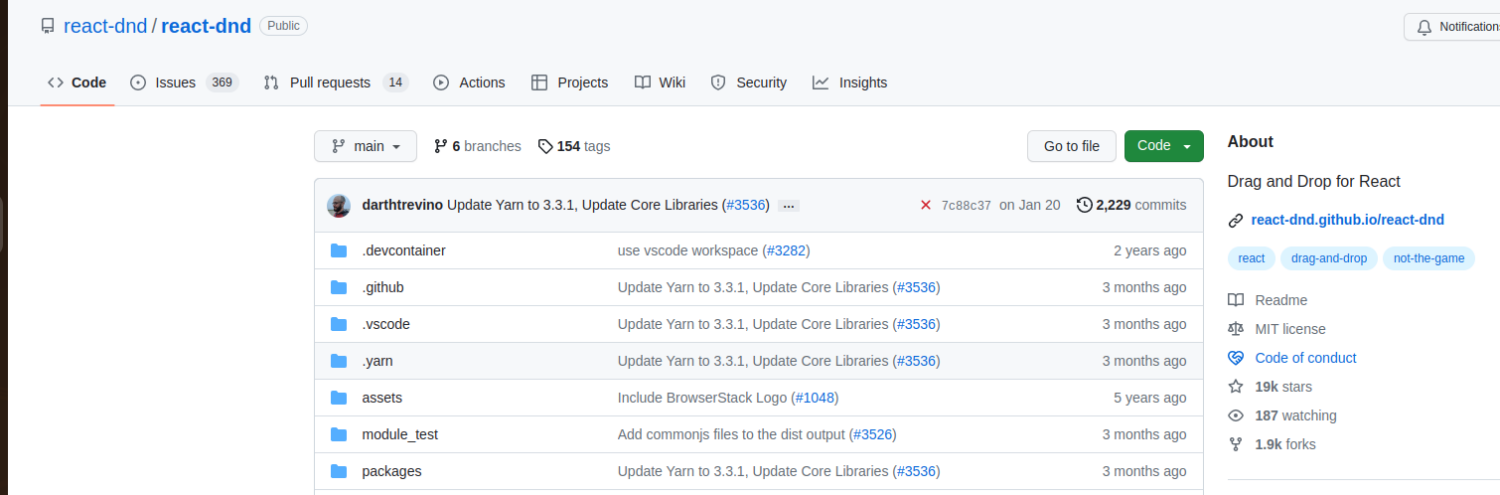
Installation;
npm install react-dnd react-dnd-html5-backend
You can import React DnD to your React component as;
import { useDrag } from 'react-dnd'
Key features
- Works with your components: This library does not provide ready-made widgets. However, it wraps your components and injects props into them.
- Extensible: You can add a custom backend based on mouse events or touch events when using React DnD library.
- Testable: You can use Jest or Enzyme to test React DnD code.
- Touch support: React DnD touch backend adds touch functionality to this library.
React DnD is a free, open-source library.
React Draggable
React Draggable is a simple yet powerful React library that makes it easy to create draggable elements.

Installation;
npm install react-draggable
To use React Draggable, import as follows on your React component.
import Draggable from 'react-draggable';
Features
- Easy to install and configure: You just need to install and import the library to get started. You can also import individual components from the library.
- Compatible with vanilla JavaScript and React: You can use React Draggable with plain JavaScript as follows;
let Draggable = require('react-draggable');
let DraggableCore = Draggable.DraggableCore;
- Compatible with other React libraries: You can use React Draggable with other libraries, such as React Grid Layout.
React Draggable is a free, open-source Drap and Drop React library.
React Dropzone
React Dropzone is a simple React Hook for creating an HTML-5-compliant drag-and-drop zone for files.

Installation;
npm install --save react-dropzone
or:
yarn add react-dropzone
You can then import this library as follows;
import {useDropzone} from 'react-dropzone';
Features
- Styling Dropzone: This library does not set any styling rules, and you can thus style your components as you see fit.
- Allows users to define acceptable file types: You can instruct the Dropzone to accept or reject certain file types by providing accept prop.
- Accepts custom validation: The validator prop allows you to specify custom validation for different files.
React Dropzone is a free, open-source Drag and Drop React library.
React Grid Layout
React Grid Layout is a resizeable and draggable grid layout for React.
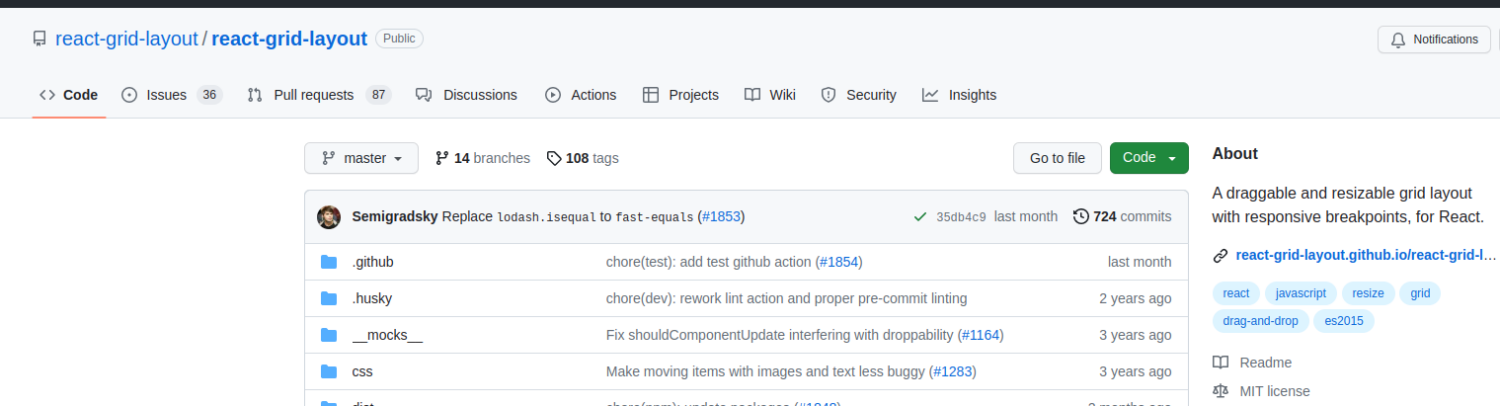
Installation;
npm install react-grid-layout
You can import this library as follows;
import GridLayout from "react-grid-layout";
Features
- Dependencies-free: React Grid Layout is built purely on React and is free of JQuery.
- Resizable widgets: On top of the drag-and-drop feature, you can also resize the components.
- Responsive breakpoints: The library provides a separate layout for every breakpoint.
- Customizable: You can add or remove widgets without rebuilding the entire grid.
React Grid Layout is a free, open-source React library.
React rnd
React rnd is a draggable and resizable component for React.
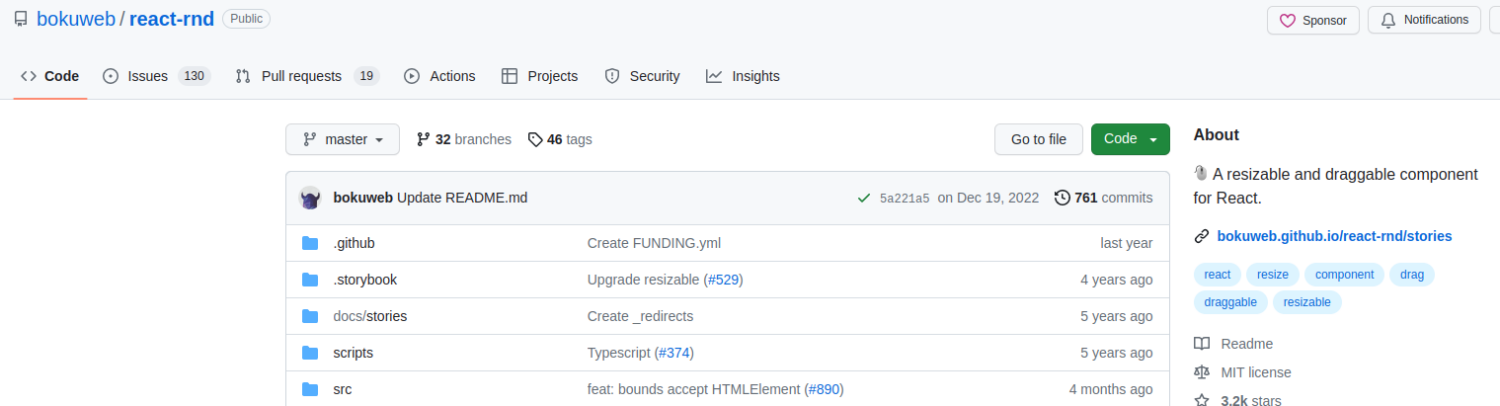
Installation;
npm i -S react-rnd
Or
yarn add react-rnd
Features
- Simple: React rnd is designed to be simple but very powerful.
- Compatible with both TypeScript and JavaScript: You can use React rnd with your app irrespective of whether you have configured it with JavaScript or TypeScript.
- Supports resizing: You can easily resize components created using React rnd to suit your needs.
React rnd is a free, open-source React library.
React Virtualized dnd
React Virtualized dnd is a drag-and-drop React framework that features inbuilt virtualizing scrollbars.

Installation;
npm install --save react-virtualized-dnd
You can import React Virtualized dnd as;
import ExampleBoard from 'react-virtualized-dnd';
Features
- Variety of components to choose from: The components are grouped into “Fixed Height”, “Variable Height” and “Groupable Droppables”.
- Customizable: You can customize the components from React Virtualized dnd to suit your needs.
React Virtualized dnd is an open-source React framework whose source code is hosted on GitHub.
React Movable
React Movable is a drag-and-drop React library for lists and tables.

Installation;
yarn add react-movable
You can import this library as;
import { List, arrayMove } from 'react-movable';
Features
- Various drag-and-drop options to choose from: The library has boilerplate codes for different types of drag-and-drop components to choose from.
- Lightweight library: React Movable does not have dependencies such as JQuery. It is less than 4kB (gzipped).
- Unopiniated styling: React Movable does not control how you may want to style your app.
- Touch support: This library helps creates apps that can be used on smartphones, tablets and any device with touch functionality.
- Written in TypeScript: You can introduce Types to your code, a functionality unavailable in JavaScript.
React Movable is a free, open-source React library.
Draggable
Draggable is a drag-and-drop React library that allows developers to create drag-and-drop events by abstracting native browser events into a comprehensive API.

Installation;
npm install @shopify/draggable --save
or via yarn:
yarn add @shopify/draggable
You can import Draggable to your React app as;
import { Draggable } from '@shopify/draggable';
Features
- Categorized components: Finding the exact component to use is easy as the components are categorized. These components are customizable.
- Compatible with major browsers: You can use Draggable with browsers such as Google Chrome, Mozilla Firefox and Apple Safari.
- Supports TypeScript: When working with this library, you can add TypeScript definitions to your code.
- Supports plugins: You can add to Draggable’s functionality with plugins such as Collidable and SwapAnimation.
Draggable is a free, open-source React library maintained by contributors.
React Drag to select
React drag-to-select is a React library that you can use to add drag-to-select functionality to your application.

Installation;
npm install --save @air/react-drag-to-select
Or
yarn add @air/react-drag-to-select
You can import this library to your app as follows;
import { useSelectionContainer } from '@air/react-drag-to-select'
Features
- Simple: This library does not select items. However, the library draws the selection box and gives you coordinates.
- Supports TypeScript: React Drag-to-select library is written in TypeScript, which means you can use it with React apps written in TypeScript and JavaScript.
- Supports testing: You can use this library with most React testing frameworks.
React Drag-to-select is a free, open-source library.
React Dragula
React Dragula is a simple drag-and-drop React library.
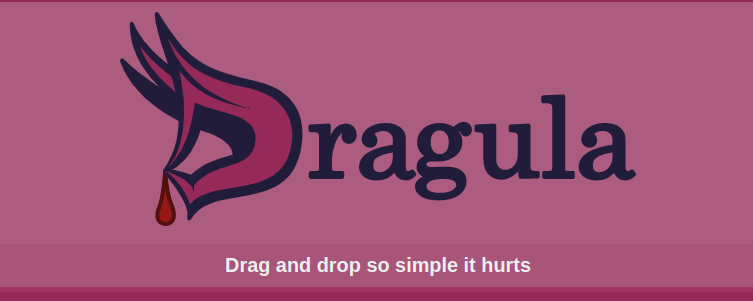
Installation;
npm install react-dragula --save
Or,
bower install react-dragula --save
Features
- Customizable: You can customize the components from React Dragula to suit your needs.
- Supports touch: You can use this library to create apps that can be used on smartphones, tablets and other touch devices.
- Lightweight: React Dragula has a small bundle size, making it perfect if you want your React app to be small.
React Dragula is a free, open-source library.
Conclusion
You now have a variety of Drag-and-Drop libraries that you can use on your next React application. The library choice will depend on the features you intend to have on your next app, taste, and preferences.
If your app is big, you may use multiple drag-and-drop libraries to cater to different needs. Most of these libraries are compatible with various React testing libraries, making it easy to ship error-free applications.