Creating PDF documents from HTML is a useful feature in several applications. Generating PDFs entirely on the frontend is even more beneficial as it allows you to simply your applications and build fully frontend applications.
By generating documents on the client side, you reduce the amount of work the server has to do. This means you will use fewer resources and save up on the reduced computing power required.
Generating documents on the client side means your applications will not require a backend. This means you can build an app with only a front end and distribute it as such.
Generating PDFs using HTML is also easier as it is easier to specify the layout of your document.
How to Convert HTML to PDF
There are multiple options for converting your HTML Document to PDF. In this section, I will demonstrate them all. Before we begin, let’s set up a basic project.
Create an HTML file with the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h1>HTML to PDF</h1>
<p>This documented was converted from HTML to PDF</p>
<script src="./script.js"></script>
</body>
</html>
This file displays some simple markup. This markup is going to be converted to PDF later. We also link a JavaScript script. This script will generate the HTML.
If you open the page in the browser, it will look like this:

Next, create the script.js file and leave it blank for now.
By Calling the Print Function
The first method, which is also the simplest method, is called the print function. This function, made available by the browser API, calls the default system library to print the HTML rendered in the page. The user can then choose the option to print the document as a PDF.
To use this method, add the following code to your script.js
function generatePDF() {
print();
}
generatePDF();
In this code, we have created a generatePDF function that calls the print function. This generatePDF function can then be called from anywhere in your code. For example, it can be attached as an event handler to the call to a print button.
In this case, we are simply calling it. This then opens up the print dialog box in the browser like so.
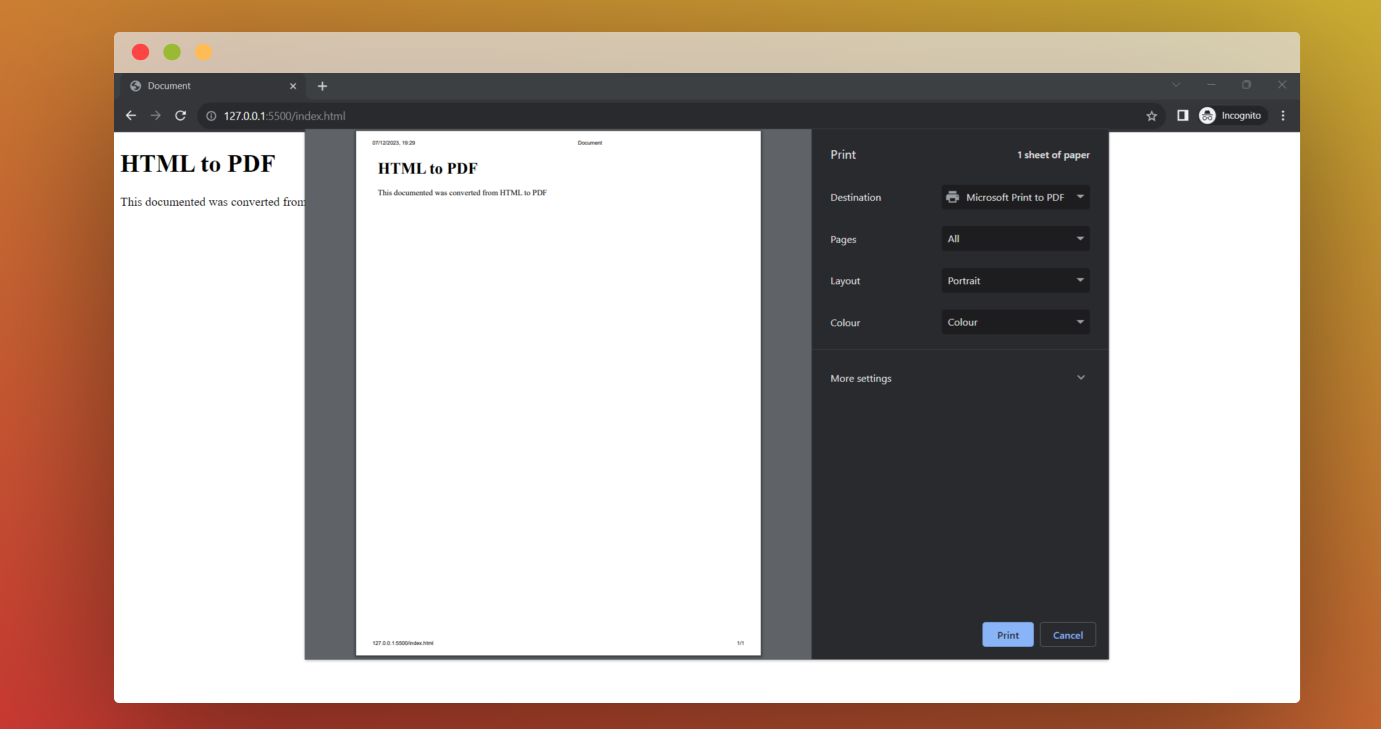
The user then has the option to specify the printer destination, of which PDF is one of the options. This will generate the PDF you want.
By using the jsPDF Library
The first method, though simple, is a little tedious. It also might not be immediately obvious to your users that you want them to print the PDF version. An easier method is to use the jsPDF library. This library lets you convert your HTML document to PDF and download it immediately.
To get started, we will have the markup from before:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h1>HTML to PDF</h1>
<p>This documented was converted from HTML to PDF</p>
<script src="./script.js"></script>
</body>
</html>
Next, we will add script tags to load the jsPDF library and other libraries it requires to work correctly before the script tag for the script.js file.
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jspdf/1.0.272/jspdf.debug.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2canvas/0.4.1/html2canvas.js"></script>
So your HTML should look like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h1>HTML to PDF</h1>
<p>This documented was converted from HTML to PDF</p>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jspdf/1.0.272/jspdf.debug.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2canvas/0.4.1/html2canvas.js"></script>
<script src="./script.js"></script>
</body>
</html>
Inside the script.js file, we can begin writing the JavaScript to generate the PDF. So, we begin by adding the function boilerplate.
function generatePDF() {
}
generatePDF();
Next, we can begin writing the code for the function.
function generatePDF() {
let pdf = new jsPDF("p", "pt", "a4");
let options = { pagesplit: true };
pdf.addHTML($("body"), options, () => {
pdf.save("myDocument.pdf");
});
}
generatePDF();
The code in the generatePDF function does the following:
It instantiates the jsPDF class. This creates a new PDF document. Next, we create an options object. In this object, we set the pagesplit property to true. This allows the PDF document to be split into multiple pages.
Lastly, we add the HTML. This is done by targeting the element whose markup we want to add to the PDF. In this case, we specified the body tag. We also pass in our options object. We also passed in a callback function, which will be executed once the PDF document has been generated. This callback calls the save method to download the document, and we supply the document’s name.
If you run the above code, you should get a Download prompt or automatic download, depending on your system settings.
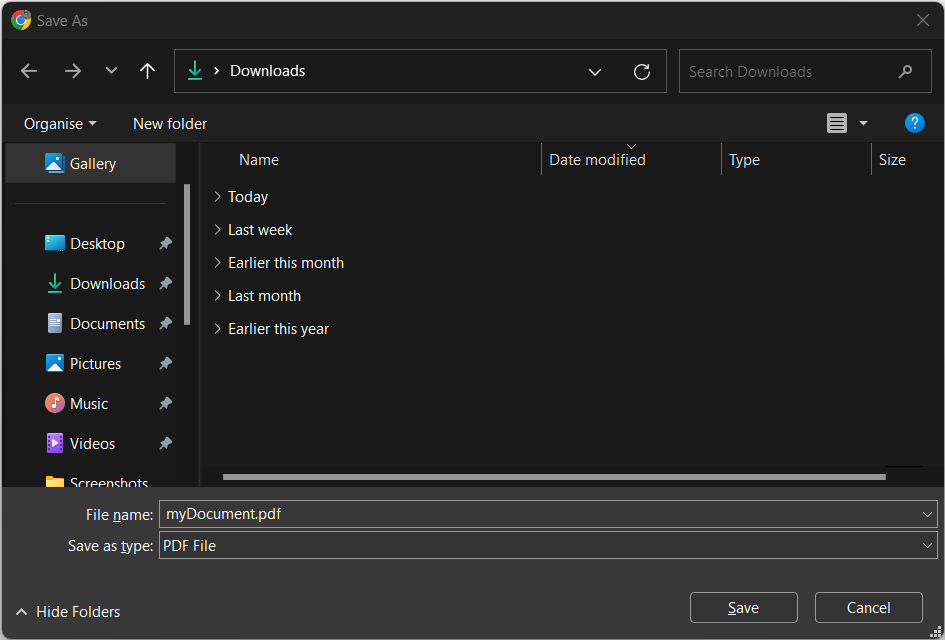
By html2pdf
The last method we will cover in this article is the html2pdf method. This is similar to the jsPDF method. This method is simpler than the previous one but offers fewer options for configuration.
To use this, let’s start with HTML boilerplate from previous examples:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h1>HTML to PDF</h1>
<p>This documented was converted from HTML to PDF</p>
<script src="./script.js"></script>
</body>
</html>
Next, add the script tag below just before the script tag for script.js. This script tag should load the jsPDF bundle.
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2pdf.js/0.10.1/html2pdf.bundle.min.js" integrity="sha512-GsLlZN/3F2ErC5ifS5QtgpiJtWd43JWSuIgh7mbzZ8zBps+dvLusV+eNQATqgA/HdeKFVgA5v3S/cIrLF7QnIg==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
Next, let’s modify the script.js file to download the PDF. Again, we start with the boilerplate code.
function generatePDF() {
}
generatePDF();
Inside the function we will add code to download the pdf
function generatePDF() {
html2pdf().from(document.body).save('myDocument.pdf')
}
generatePDF();
This code calls the html2pdf function, which returns an object that has a from method. We then call this from the method and pass a reference to a DOM element from which we would like to create the PDF document. Next, we call the save method on the returned object. We pass in the file name to the save method.
Running this code should prompt you to download the PDF file.
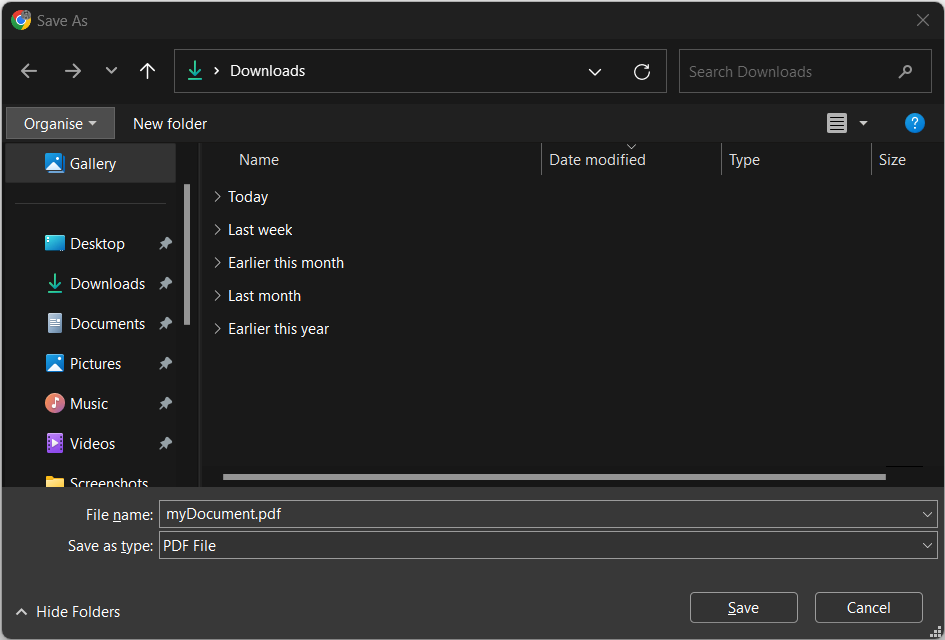
Wrapping Up
In this article, we covered three of the most popular methods for converting your HTML markup to PDF from the front end. Doing this has numerous advantages, including allowing you to build fully frontend applications.
However, the PDFs generated by methods 2 and 3 are first rendered as images and then added to the PDF. This means the text cannot be highlighted. This can be an advantage or disadvantage.