Imagine 20 names and their phone numbers written in a simple Word doc. Hard to follow, but still, you might manage to read. Now imagine 200 names, but this time their serial numbers and addresses, along with phone numbers in plain text. Clumsy right?
Don’t you think it would be more clear if we could see this data in a structured format? Yes right! That’s why data scientists or analysts use formatted data for their analysis. Two such formats to store or work with data are JSON and CSV.
JSON are lightweight files known for data exchange between applications or servers. They store data in key-value pairs.
While CSV files are comma-separated values to store big data. Though they are comma-separated values, you often find them in tabular structure while using Python for analysis.
Why Convert JSON to CSV in Python?

Faster Data Analysis: Python operations on CSV files are faster. You can quickly view, sort, filter, and trim data faster when in CSV format. Also, Python has a library called Pandas for efficient data analysis of CSV files.
Support for Various Data Processing Tools: Though JSON files are lightweight and easy for data exchange, CSV files are more comfortable to visualize and analyze using databases like spreadsheets and programming languages like Python.
Memory Efficient: JSON format includes metadata, putting some load on memory, whereas CSV is just comma-separated values with no additional punctuations or metadata. Plus, parsing JSON data requires more memory than CSV.
So, compared to JSON, CSV format is more memory efficient in storing and processing large datasets.
Human Readable: CSV format is well suited for manual inspection. When opened in tables or spreadsheets, you can easily read and understand the CSV data, while JSON format is somewhat complex to read, especially when it includes nested objects.
Performance: Parsing and processing CSV files is faster and demands less memory compared to JSON files, resulting in optimal performance.
How to Convert JSON to CSV in Python?
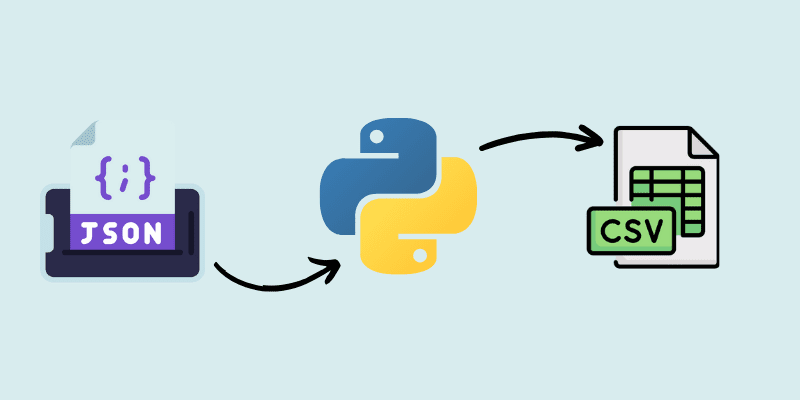
Here are the steps to convert JSON to CSV in Python.
Step 1: Import JSON and CSV built-in modules.
import json
import csv
Step 2: Let’s assume that we have the JSON data in a file named json_data.json. Read this data into a variable using load function.
with open('json_data.json') as data:
json = json.load(data)
Step 3: Create a csv file with JSON headers as column names and corresponding values in the cells.
headers = json[0].keys()
with open('data.csv', 'w', newline='') as csv_file:
csv_writer = csv.DictWriter(csv_file, fieldnames=headers)
csv_writer.writeheader()
for row in json:
csv_writer.writerow(row)
How to Convert JSON to CSV Using Pandas in Python?
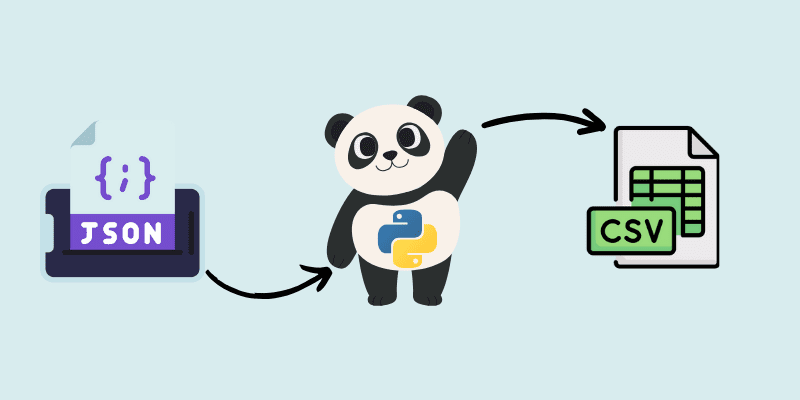
You may write your own custom code for the conversion using JSON and CSV built-in libraries as shown above, but Pandas is your friend. It makes the conversion even simpler with just two lines of code.
For those don’t know Pandas, it is Python’s library to manipulate and analyze large data. Here is how you can easily convert JSON to CSV using Pandas.
Step 1: To convert JSON files to CSV, you first need to import Pandas in Python.
Import pandas as pd
Step 2: Load the JSON data into Pandas DataFrame.
data = pd.read_csv('json_data.json')
Step 3: Write the data to CSV file.
data.to_csv('csv_data', index=False)
The file named ‘csv_data’ will be created in the current working directory to store the converted CSV data.
“index = False” here excludes the index column in the CSV file.
That’s it, it is this easy to convert JSON to CSV using Pandas.
Things to Consider While Converting Large JSON Files to CSV
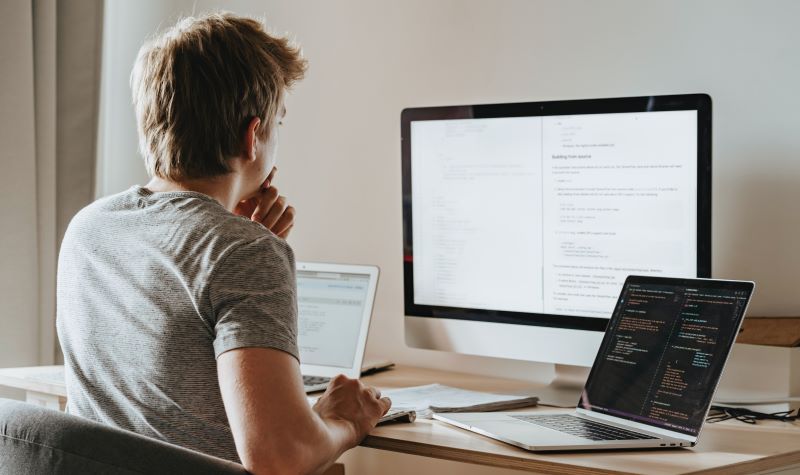
- Memory – The very first issue you usually face with large datasets is memory. If you try loading entire data to a CSV file in one go, you might run into memory issues. So, process the large data into CSV in chunks to avoid memory issues.
- Remove Redundancy – Having the same redundant data multiple times in the dataset just increases the size but doesn’t add any value to the data. So, remove the redundant data from your dataset. This way, you can reduce the size of the data file, resulting in faster conversion.
- Use Libraries – If the data is small, you can write a custom Python code to convert it into CSV, but in the case of large data, you should use a Python library like Pandas to efficiently convert JSON to CSV.
- Backup – Have a backup of your data while converting large JSON files to CSV. This helps you get back to the original data if something goes wrong with the conversion.
Potential Errors that May Arise During Conversion
#1. Unicode Error
You would encounter this error when you write special characters or non-ASCII characters to a CSV file from JSON data.
To fix this, you should specify an appropriate encoding in the open function used when converting JSON to CSV files.
You can do this by adding a parameter called encoding to the open function, as shown below:
with open('data.csv', 'w', newline='', encoding='utf-8') as csv_file
#2. Value Error: Expected object or value
This error pops up when there is an issue with the content of the JSON file, mainly when the function is unable to parse the JSON object or file.
So, ensure your JSON data is well-formatted and follows JSON syntax.
Moreover, if your JSON data contains nested objects like arrays, make sure the JSON objects are enclosed in [..] and the arrays are wrapped in [..]. Also, ensure the data is flattened using “JSON.normalize” before converting nested JSON to CSV.
#3. CSV Headers
One of the main differences between JSON and CSV is that JSON data doesn’t have headers like CSV. So, handle the CSV headers well while converting JSON to CSV. You can give them values or leave them empty as you wish.
But if you are giving headers to the CSV file, ensure their data type matches with the actual data in the file.
Conclusion
Converting JSON to CSV is easy when done in Python. Even simpler if we use the Pandas library.
So, this article shows you how to convert JSON files to CSV using Python. Also, we have shown you how to use Pandas to efficiently convert JSON to CSV.
Followed by some important things to keep in mind for a smoother conversion process and common errors you might encounter during conversion.
You may also check out converting JSON files to Excel.
-
EditorNarendra Mohan Mittal is a senior editor at Geekflare. He is an experienced content manager with extensive experience in digital branding strategies.