Python is an interpreted, general-purpose programming language that has been growing in popularity and use among the development community.
In fact, the 2022 Stack Overflow Developer Survey found that Python is the fourth most-used programming language by professional developers and the third most-used language by those learning how to code.
Python’s popularity and wide use can be attributed to its simplicity, clean syntax, and ease of use which makes it easy to learn even for absolute beginners.
Python has wide applications ranging from web development, machine learning, data science, artificial intelligence, game development, and robotics, among many others.
When learning how to code in Python, the typical progression starts with learning fundamental concepts such as working with variables, different data types, conditionals, loops, functions, and file handling.
Once intermediate concepts have been properly understood, the next step should be learning how to build graphical user interfaces using Python GUI libraries.
A Graphical User Interface(GUI) is a type of user interface that allows users to interact with applications through graphical elements such as windows, icons, buttons, menus, and dialog boxes.
Compared to a Command Line Interface(CLI), where users interact with an application by typing commands into the terminal, a GUI is a more user-friendly and intuitive alternative. It also makes applications easier to use among nontechnical users.
To build graphical user interfaces in Python, you need to utilize Python’s GUI Libraries. Whereas you can hard code the graphical elements yourself, it makes more sense to use prepackaged GUI Libraries.
Libraries contain prewritten reusable code that can be used to provide useful features, optimize tasks or solve problems that may arise during development.
In this case, Python GUI libraries provide prebuilt tools, components, and features that make the process of building user-friendly interfaces easier and faster.
Benefits of the Python GUI Library
Using the Python GUI library when building graphical user interfaces is very beneficial. Some of the benefits include:

Simplifies the development process
Python GUI library simplifies the process of developing graphical user interfaces by providing prebuilt GUI components. These components provide a high level of abstraction, such that developers don’t need to know how to develop the component itself. All they need to know is how to use it in their development process. This, in turn, makes building GUIs easier.
Allows for faster development
Since GUI libraries come with prebuilt components that are ready for use, they help make the development process faster as developers don’t have to worry about building their own components. It can also come in handy when you need to prototype an application within a short time.
Provides a rich set of components
GUI libraries offer a rich set of prebuilt graphical user interface widgets. Some of these widgets include icons, buttons, labels, text inputs, dropdowns, checkboxes, progress indicators, resizable window borders, and forms, among others. This, in turn, allows developers to create more interactive, user-friendly, and feature-rich graphical user interfaces.
It can be integrated with other libraries
Python GUI libraries are built in such a way that they can be integrated with other Python Libraries. For instance, you can integrate a GUI library with other Python libraries such as NumPy, Flask, and Tensorflow to create robust and comprehensive applications.
Comes with event handlers
Interactive applications need to handle events from users. In that regard, Python GUI libraries can handle event-driven programming allowing developers to respond to users’ actions such as scrolling, clicking buttons, typing into text inputs, and mouse actions, among others.
This makes it easier for developers to implement interactive user interfaces that can respond to users’ actions and engage users of an application.
When it comes to building graphical user interfaces, Python GUI libraries handle all the heavy lifting allowing developers to focus their efforts on more important and unique aspects of their GUI without having to worry about building GUI components themselves.
How Python GUI Library Enhances User Interface
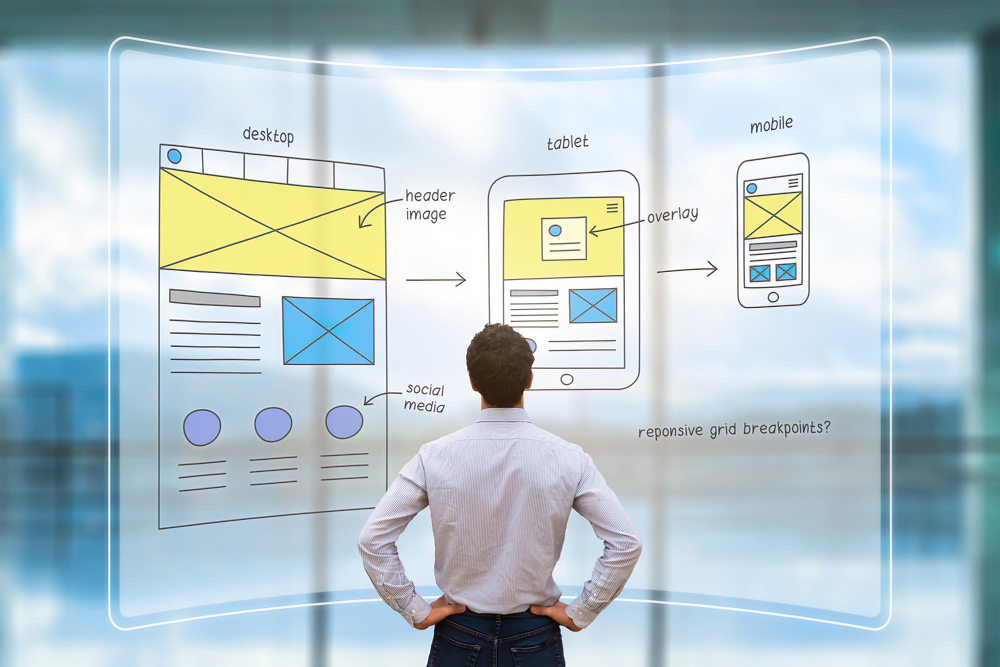
Regardless of how good an application is, the application’s user interface will be a key determinant of its reception by intended users, whether users enjoy using the application, and how well users can utilize the application.
A good user interface needs to provide users with a seamless, intuitive, and enjoyable interaction that makes an application usable, valuable, and desirable among users. Developers can achieve all this by utilizing Python GUI libraries.
First, Python GUI libraries come with layout managers for arranging and positioning UI elements on the screen. Proper layout and placement of components make user interfaces both usable and their components findable.
The fact that GUI libraries come with prebuilt components also serves to enhance the user experience by providing a robust set of components that can be used to make it easier for the user to interact with an application.
Additionally, Python GUI libraries allow for theming and styling of GUI components. By using a GUI Library, developers can change the colors, fonts, appearance, and size of elements on the screen.
This allows the creation of visually applying designs that are enjoyable to use and aligning user interfaces with the general brand colors and themes to create consistency in products.
Python GUI libraries also provide the ability to integrate multimedia components such as videos and images to further enhance the appearance and visual appeal of a graphical user interface.

Bearing in mind that an application might be used by users with disabilities, Python GUI libraries provide accessibility features that account for such users.
Accessibility features such as high contrast mode, support for screen readers, provision of alternative text for visual elements, and keyboard navigation enhance the user interface of applications allowing people with disabilities to use the applications.
Python GUI libraries are thus an important component when building user interfaces in Python. Here are the top Python GUI libraries to help you build better user interfaces faster:
PyQT
PyQt is a set of Python bindings for the Qt application framework that runs on Windows, macOS, Linux, iOS, and Android. A Python binding allows developers to call functions and pass data from Python to libraries written in languages such as C or C++.
Qt is a GUI framework written primarily in C++, and thus PyQt exposes the toolkit to Python developers.
PyQt has a comprehensive set of widgets and controls ranging from basic widgets such as buttons, labels, checkboxes, and radio buttons to more advanced components like multimedia players, graphs, and tables.
PyQt allows developers to fully customize the prebuilt components to their liking and also create their own custom widgets allowing room for lots of creativity and possibilities when developing user interfaces.
Its deep integration with Qt has the benefit of ensuring that GUIs developed using PyQt have a native look and feel across different operating systems. PyQt is ideal for developing complex GUIs for data visualization tools, interactive multimedia software, desktop applications, and complex business or scientific applications.
DearPyGui

DearPyGui is a powerful, cross-platform, GPU-accelerated GUI framework for Python that allows for complete style and theme control. The framework is written in C/C++, allowing the framework to be used to create high-performant Python applications.
As a GUI framework, DearPyGui comes bundled with traditional GUI Elements such as menus, buttons, radio buttons, and a robust collection of dynamic plots, drawings, tables, a debugger, and multiple resource viewers. The framework also comes with a variety of methods needed to create a functional layout.
Being both a powerful and an easy-to-use framework with GPU-based rendering, DearPyGui is ideal for creating both simple interfaces for Python scripts and very complex user interfaces.
The framework is well-suited for developing GUIs for applications that require fast and interactive interfaces in gaming, data science, and engineering applications.
PySimpleGUI
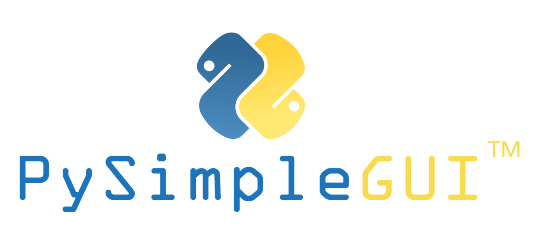
PySimpleGUI is a wrapper package that currently supports four Python GUI frameworks namely: tkinter, Qt, WxPython, and Remi. By wrapping around the four frameworks, PySimpleGUI encapsulates the specific objects and code used by the GUI framework you’re running PySimpleGUI on top of.
PySimpleGUI also implements most of the framework’s boilerplate code for you so that your PySimpleGUI code is much simpler and shorter compared to writing code directly in the underlying framework.
According to its documentation, a PySimpleGUI program may require ½ to 1/10th amount of code to create an identical window using one of the frameworks directly. PySimpleGUI makes it easier to migrate to different GUI frameworks.
PySimpleGUI is ideal for those learning how to create Python GUI, as it makes it easier to build GUIs. However, PySimpleGUI is only ideal for simple GUIs and is not the best option in case you want to build more complex GUIs.
Kivy

Kivy is an open-source, cross-platform Python GUI framework written mostly in Python and Cython. Aside from being highly customizable, Kivy allows for writing highly reusable code that can be deployed on Windows, macOS, Linux, Android, and iOS.
Kivy is designed to be highly customizable and easy to use, allowing for quick and rapid prototyping of GUIs for applications. Although Kivy has cross-platform support, the framework is catered for developing applications that make use of multi-touch user interfaces.
All Kivy widgets are built with multitouch support. Its cross-platform nature, coupled with its multitouch support, explains why most real-world Kivy applications are Android and iOS. If you’re building touchscreen interfaces for Android or iOS devices, this should be your go-to Python GUI framework.
Tkinter
Tkinter is the default standard GUI package for Python. Since it is part of the Python standard library, Tkinter has grown in popularity and use.
This has been beneficial to the library as its large community of users has ensured that the library has clear and rich documentation allowing even complete beginners to easily learn the basics of how to use the library to build GUIs. Additionally, Tkinter easily integrates with other Python libraries.
Tkinter comes with a wide range of pre-built components such as buttons, labels, menus, text boxes, checkboxes, progress bars, and tabbed views, among others.
Coupled with the fact that Tkinter has cross-platform compatibility, developers can use the library to build rich GUIs that work on major operating systems.
Tkinter is ideal for rapidly prototyping user interfaces and building simple GUIs for small applications. It is, however, not ideal for building more complex GUIs with lots of dependencies.
Toga

Toga is a Python native, OS native cross-platform GUI toolkit that is still in its early development but comes with lots of features and tons of base GUI components. Toga, which requires Python 3.7 or higher, is built with the understanding that mobile computing has become very important.
Toga thus aims to be the go-to Python GUI framework for Python programming on mobile platforms and allow for cross-platform mobile coding.
Unlike other GUI frameworks that achieve cross-platform compatibility by applying an operating system-inspired theme on top of generic widgets, Toga uses native system widgets on each operating system. Using native widgets makes GUIs built using Toga faster and allows developers to build cleaner native applications.
Toga provides an API that exposes a set of mechanisms for achieving UI goals and also abstracts the broader concepts of building GUI apps. This way, every Toga application comes with the basic set of menu options, all in the places you’d expect to find them in a native application.
Qt for Python
Qt for Python is the official set of Python bindings for the Qt framework, which is written by the developers of the Qt framework developed using C++.
Qt for Python has a large community of users and large companies that rely on it to create advanced graphics and complex user interfaces. Qt for Python shines in creating complex, visually stunning, and high-performance applications which run on various platforms.
Qt for Python comes with ready-made controls and widgets ranging from basic GUI components to advanced GUI components. Developers can also create complex data visualizations using Qt for Python and work with 2D and 3D graphics in their development.
Whereas learning how to fully utilize its features in creating complex GUIs may take some time, beginners can still benefit from using Qt for Python in creating simple GUIs before they can move to more advanced GUIs.
Conclusion
Python has a lot of GUI libraries and Frameworks that can be used in building simple GUIs to complex, visually stunning GUIs that run across various platforms.
Depending on your level of expertise working with Python GUI and the GUI you want to build, a variety of libraries might be ideal. For instance, absolute beginners wanting to build simple user interfaces might find PySimpleGUI easier to pick and use.
On the other hand, more experienced developers looking to build more complex, responsive, and visually stunning user interfaces may benefit from using PyQt or Qt for Python.
Therefore, when building GUI, consider the complexity and features of your user interface and your experience working with GUI before settling on a Python GUI for your project.
You may also explore some Python linter platforms to clean up your code.