Bootstrap is everywhere, but it’s not always the right tool for the job. Here are some great alternatives!
If you check the source code of a website’s front end at random these days, chance are you’ll find Bootstrap underneath.
We’ve all become so used to concepts like container-fluid, row, col-sm-6, etc., that it’s hard to imagine that any other style of front-end development is even possible. And so when we have to build the next project, we reach for Bootstrap unconsciously. That said, the popularity doesn’t make Bootstrap a good fit for all projects and needs.
In fact, for really lean frontends, loading all the Bootstrap CSS and JS can cause major bloat.
This article has two purposes:
- Provide non-Bootstrap-like live alternatives to Bootstrap
- Explain why you might want to consider these alternatives over Bootstrap
I think the explanation part is really important because in most cases, people don’t even realize they have a problem or that they’re making their job harder by picking up Bootstrap. Finally, please note that this is not an anti-Bootstrap post by any means.
I love Bootstrap 4 and use it whenever I can. But, then, I’m an individual developer who has to think of using the most popular solution; also, I’m not a UI developer per se, so I don’t worry about too many things when building out my front-ends.
And with that, let’s have a look at what alternatives we have.
Flexbox Grid
Think about it for a minute: the biggest reason you started using Bootstrap and are still using it is its grid system. Sure, it took some getting used to the row
, col-md-6
, etc., classes, but now it’s second nature to think of a layout in terms of rows, columns, offsets, etc.
And if you’re honest with yourself, you’ll find that everything else in Bootstrap is a bit of a chore to work with.
There are tons of classes to remember, whether you’re doing forms, navigation, buttons, tables, or anything else. If you’re like me, you’ve still not gotten used to all the classes and what they do, and you often use Bootstrap only for the grid and write all the other CSS yourself.
If yes, you could do a lot better with Flexbox Grid.

The Flexbox Grid, as the name suggests, is a grid system based on the CSS Flexbox properties. However, unlike the CSS technique, all the complexity is nicely abstracted away so that you focus only on placing elements the way you want.
The best part is that all the code and class names mimic what you’d want in Bootstrap 4, which means switching between these two tools requires zero mental friction. For instance, here’s what code for the “space around” looks like in the Flexbox Grid:
<div class="row around-xs">
<div class="col-xs-2">
<div class="box">
around
</div>
</div>
<div class="col-xs-2">
<div class="box">
around
</div>
</div>
<div class="col-xs-2">
<div class="box">
around
</div>
</div>
</div>
The minified CSS file for this grid system is a mere 10.7 KB saving you several hundred KB in the final download size. These days the Flexbox Grid is my favorite as I don’t want to fight Bootstrap to customize it fully. I like to start with vanilla elements and style them myself, using the Flexbox Grid wherever I need to.
Learn Flexbox here, online.
PureCSS
Wouldn’t it be nice if Bootstrap was split into modules and you could import only the module you needed?
Well, PureCSS has gone ahead and done just that — it’s a set of modules covering different functional areas of a website. You can choose to download one or all, and yet the download size won’t exceed 3.7 KB!

Yes, you read that right.
All the modules when bundled together and gzipped are 3.7 KB, although individually they amount to more. The grid module is just 0.8 KB, while the Base module is 1.0 KB. The team behind PureCSS says that it was built entirely with mobile devices in mind, and so every line of CSS was carefully scrutinized for efficiency before being included.
So let’s say you need just the grid and forms module. Well, just download these two (along with the Base module), and you’d be done in less than 3.4 KB! No need to include the CSS from Buttons, Tables, and Menus modules if you’re not going to use them.
PureCSS has its classes, though, and the resulting code doesn’t mimic the Bootstrap you might be very used to:
<div class="pure-g">
<div class="pure-u-1 pure-u-md-1-2 pure-u-lg-1-4">
<div class="l-box">
<h3>Lorem Ipsum</h3>
</div>
</div>
<div class="pure-u-1 pure-u-md-1-2 pure-u-lg-1-4">
<div class="l-box">
<h3>Dolor Sit Amet</h3>
</div>
</div>
<div class="pure-u-1 pure-u-md-1-2 pure-u-lg-1-4">
<div class="l-box">
<h3>Proident laborum</h3>
</div>
</div>
<div class="pure-u-1 pure-u-md-1-2 pure-u-lg-1-4">
<div class="l-box">
<h3>Praesent consectetur</h3>
</div>
</div>
</div>
You’ll notice that there’s no 12-column grid anymore. PureCSS has its grid system that specifies how much width a column should take. So, pure-u-lg-1-4
means that this element should take 1/4 or 25% of the available width on large screens. Widths as multiples of 1/5 are also available.
All in all, PureCSS is a liberating and amazing CSS tool (framework?) that you can pick and choose from as needed. That said, it does come with a fair amount of buy-in and a learning curve as you need to learn a new (slightly different) way of doing things.
PureCSS also has its own classes and default styling, so in that, it’s not too different from Bootstrap.
Zimit
The Zimit framework is kind of an odd-man-out in this list. Yes, it’s a framework for building UIs, but it’s aimed at particular types of UI: mockups.
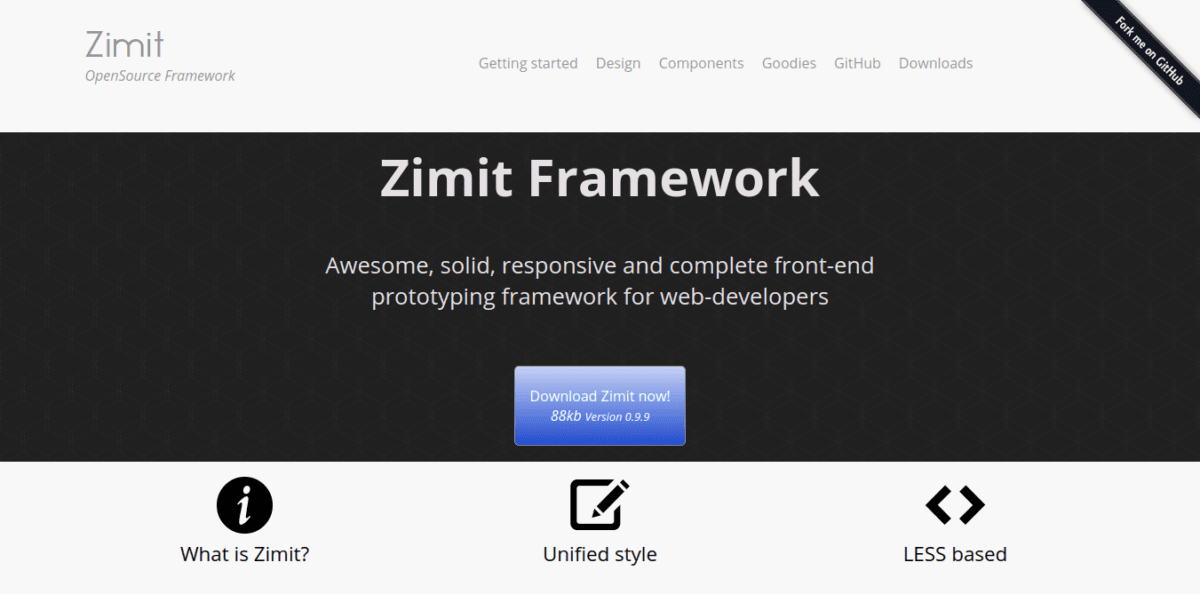
There are times when you have to develop the front-end to show the functioning of the product. The ideal way to do this, of course, would be to get a UI designer/developer involved and create the mockups on one of the advanced wireframing tools (Moqups, Blasmic, etc., come to mind).
The pages would be pixel-perfect, the color scheme sleek and well-chosen, and the pages would be open for participation, reviews, comments, etc.
But real life is not ideal, and often you’re the only man on the job and must wear all the hats and get the job done. At those times, you want a framework that:
- Allows you to code in HTML/CSS
- Is lightweight
- Has an extensive collection of fundamental components
- Has a decent and consistent style language
- If possible, resembles the “greyish” tone of wireframe design
- Is easy to learn
- Has some CSS preprocessor built-in
Zimit checks all these boxes. It’s just 84 KB in size and has a wide range of components to choose from. Here are some examples that I found really appealing, as coding them on your own will take a lot of time.
Tree view

Breadcrumb

Tabs

There are many more goodies to explore. Check them out here.
Let’s look at what the code looks like. Here’s how you’d use the grid system in Zimit:
<div class="row">
<div class="c12">
<div class="row">
<div class="c4">4 columns</div>
<div class="c4">4 columns</div>
</div>
<div class="row">
<div class="c4">4 columns</div>
<div class="c4">4 columns</div>
</div>
</div>
</div>
The “c” here stands for “column,” so “c4” means a column that spans four units (the grid being 12-size, just like Bootstrap’s). Very similar to Bootstrap, and very intuitive, in my opinion.
All in all, Zimit is a complete and easy framework for developing UI prototypes that are responsive and look good quickly. It’s better than Bootstrap (when it comes to prototyping) because Bootstrap is a much larger download and the resulting design is, well, tacky.
Tailwind
Tailwind CSS creates utility classes instead of pre-defined component styles.
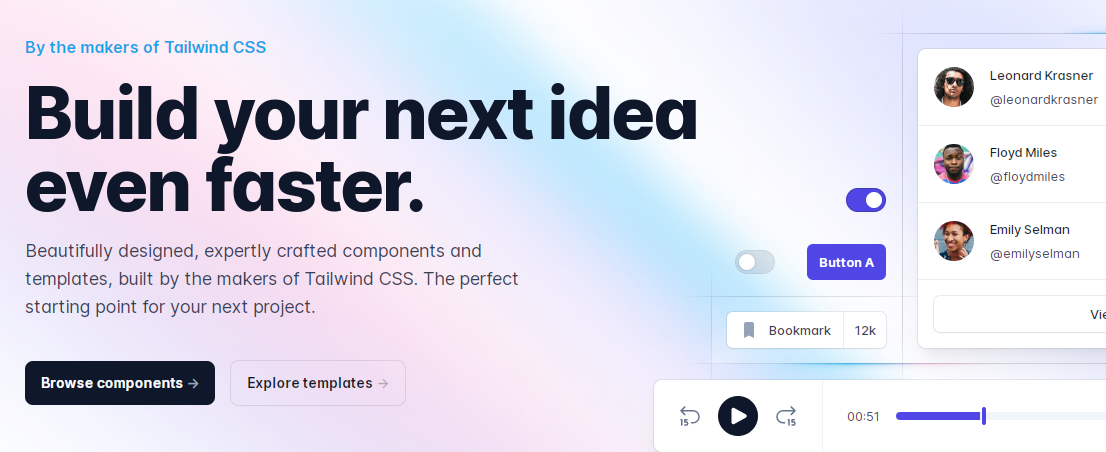
This framework allows developers to use utility classes to control shadows, typography, layout, spacing, and more, creating custom component designs on HTML files without writing code.
You can create a simple form that allows users to opt-in as follow;
<div>
<form class="m-4 flex">
<input class="rounded-l-lg p-4 border-t mr-0 border-b border-l text-gray-800 border-gray-200 bg-white" placeholder="email address"/>
<button class="px-8 rounded-r-lg bg-green-400 text-gray-800 font-bold p-4 uppercase border-yellow-500 border-t border-b border-r">Subscribe</button>
</form>
</div>

You can customize different things such as text color, background color, and border size to suit your needs.
Why Tailwind CSS?
- Low specificity: Tailwindโs selectors are less specific, making them less likely to override or conflict with other styles. You can thus write less code when using Tailwindโs utility classes and still produce maintainable code.
- Opinionated and flexible: Tailwind is designed to strike a balance between a customizable and flexible framework. This framework recommends using a consistent color palette, spacing scale, and typography. However, you can still customize some defaults to suit your needs.
- Just-In-Time (JIT) improves build time: The JIT feature offers an efficient and faster way to generate the final CSS code. With this feature, Tailwind only generates styles that are used in your HTML code. Basically, whenever you load a web page, your browser will send a request to the JIT server, which will generate an optimized CSS code for that specific file and send it back to the browser.
Tailwind CSS is suitable if you are looking for a framework with less specificity, you want to create custom designs and still enjoy flexibility.
Bulma
Bulma is an open-source UI framework based on Flexbox. The framework has a variety of ready-to-use frontend components that you can combine to create visually attractive and responsive interfaces.

The ease of configuration is one of the reasons why Bulma is gaining popularity among UI developers.
To demonstrate how it works, we will create several tabs using this code;
<div class="tabs">
ย ย <ul>
ย ย ย ย <li class="is-active"><a>Pictures</a></li>
ย ย ย ย <li><a>Music</a></li>
ย ย ย ย <li><a>Videos</a></li>
ย ย ย ย <li><a>Documents</a></li>
ย ย </ul>
</div>
The rendered page will appear as follows;

You can go ahead and customize the tabs as you see fit. For instance, you can change the active links to inactive, center the tabs, change the text on the tabs, and more.
Why Bulma?
- Bulma has a 12-column grid system, allowing you to divide your page into equal-width columns. The beauty is that these grids auto-adjust themselves based on the screen size.
- If you are looking forward to customizing your application, Bulma is perfect since it comes with a few defaults.
- This framework also offers much flexibility since it is based on Flexbox.
- Bulma is lightweight, as it uses minimal JavaScript resulting in smaller apps.
Bulma suits developers looking for a UI framework that is simple yet powerful. The modern touch of Bulmaโs components suits designers who donโt want to use generic and old-school Bootstrap designs.
UIkit
UIkit is a lightweight and modular framework for developing powerful web interfaces. You can use this framework with CSS, Less, and Sass. This will be the ideal Bootstrap alternative if you want a platform with solid HTML, CSS, and JavaScript components.

To use UIkit in your code, include its CDN link in the <head>
section. You can as well compile the source code from GitHub.
Once you are done with the installation, you can start using various components. For instance, you can add a uk-button
component as follows;
<button className="uk-button uk-button-primary">My Button</button>

Why UIkit
- Modular architecture: The components from UIkit are self-sufficient. Thus, you can include only what you need in your app. Such an approach reduces the size of your JavaScript and CSS files.
- Built-in JavaScript plugins: You can expand the functionality of UIkit through its JavaScript plugins, such as tabs, modals, and sliders.
- Customization: The availability of Sass variables and mixins gives more room for customization. You can thus come up with unique designs that match your app needs.
- UIkit auto-complete tools for your editor: You can add UIkit plugins or code snippets to your favorite code editor, such as Visual Studio Code, Sublime Text 3, or Atom, and get a helper in generating UIkit classes and mark up.
UIkit will be a perfect option for developers looking for a modern and distinct design. UIkit autocomplete for your editor
HTML KickStart
In most of the projects you build, speed is critical. The biggest hurdle to speed in web development is the front-end part, and the biggest “delayer” in front-end development is the need to code elegant-looking, interactive components.
Since there are many ways in which a component can behave, and there are many screen sizes to manage, coding, and managing components can become a nightmare for the developer.
HTML KickStart offers an alternative.

Put simply; it’s a collection of really sleek components that you can just drop into your projects and cut down the development time drastically. Here are some nice components I found:
Dropdown

Buttons

Tabs (centered and with icons)

Materialize
If you like Bootstrap for the fact that it has a readymade solution for all common web design problems, but you’re a fan of Material design style, you should try out Materialize.

Materialize is mostly just like Bootstrap — 12-point grid system, offsets, and familiar components like forms, cards, etc. However, it does have certain goodies that can appeal to many.
Push-pull
The push/pull feature of Materialize CSS allows you to reorder columns. This is reminiscent of the new CSS Grid standard, where the layout is different from the element order.
<div class="row">
<div class="col s7 push-s5"><span class="flow-text">This div is 7-columns wide on pushed to the right by 5-columns.</span></div>
<div class="col s5 pull-s7"><span class="flow-text">5-columns wide pulled to the left by 7-columns.</span></div>
</div>
This results in the following:

You’ll notice that the columns have switched places, which is something perhaps unachievable in traditional Bootstrap-based CSS.
JavaScript goodies
There are quite a few JavaScript features and effects that ship with Materialize. Tooltips, Toasts (Android-like ephemeral notifications), Parallex, Pushpin, etc., are some of them.
One really amazing effect I liked is FeatureDiscovery, which basically allows you to highlight an element on the page on some event (say, button press) to bring the user’s attention to that element. It’s hard to describe it in words fully, so head over to https://materializecss.com/feature-discovery.html to see what I mean.
All in all, Materialize is a great alternative to Bootstrap or for those looking to adopt a full-featured Material CSS framework.
Conclusion
Bootstrap is synonymous with responsive design. It was Bootstrap that popularized the term “mobile-first design” and showed how it could be done. But while Bootstrap gets the job done most of the time, just getting the job done isn’t always enough.
If you feel like Bootstrap is restricting you and that your needs are special, one of the options listed here will help.
You may also read how to add Bootstrap to Angular.